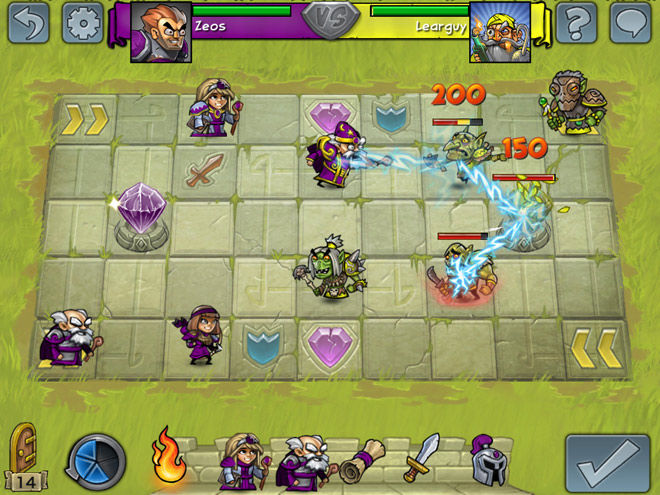
A turn-based battle system is a popular mechanic in many RPG-style games, where each character takes turns to attack or defend in a combat scenario. This tutorial will cover the basic mechanics of a turn-based battle system, including how to set up the scene, create the battle logic, and animate the battle actions.
Setting up the Scene
To set up the scene for our turn-based battle system, we will need to create a few game objects and components. First, we will create a player object and an enemy object, both of which will have a collider and a script attached. The script will contain the character's stats, such as health points, attack power, and defense power.
Next, we will create a turn manager object, which will manage the flow of turns between the player and the enemy. The turn manager will have a script attached that will handle the turn-based logic, such as determining whose turn it is, and what actions they can perform during their turn.
Finally, we will create a UI canvas that will display the battle actions and character stats. The UI canvas will have several UI elements, including health bars, attack buttons, and end turn buttons.
Creating the Battle Logic
To create the battle logic, we will start with the turn manager script. The turn manager will keep track of whose turn it is and what actions they can perform during their turn. For example, during the player's turn, they can choose to attack or defend. During the enemy's turn, they can choose to attack the player.
To determine whose turn it is, we will use a simple boolean variable called isPlayerTurn. When the battle starts, we will set this variable to true, indicating that it is the player's turn. During the player's turn, we will display the attack and defend buttons on the UI canvas, and wait for the player to choose an action.
When the player chooses an action, we will use a switch statement to determine what action they chose. If they chose to attack, we will calculate the damage based on the player's attack power and the enemy's defense power, and subtract that amount from the enemy's health points. If they chose to defend, we will increase the player's defense power for one turn.
After the player's turn is complete, we will set the isPlayerTurn variable to false, indicating that it is now the enemy's turn. During the enemy's turn, we will simply calculate the damage based on the enemy's attack power and the player's defense power, and subtract that amount from the player's health points.
Another way to keep track of whose turn it is, instead of using boolean isPlayerTurn, we can use an enum. Using an enum can be a good alternative to using a boolean to keep track of whose turn it is. In fact, using an enum can be a more flexible solution, especially if you plan on having more than two states in your turn-based system, such as "waiting for player input" or "animation in progress".
To use an enum, you can define a new enum type called TurnState, which will contain all possible states that the game can be in during a turn. In this case, we will have two states: PlayerTurn and EnemyTurn.
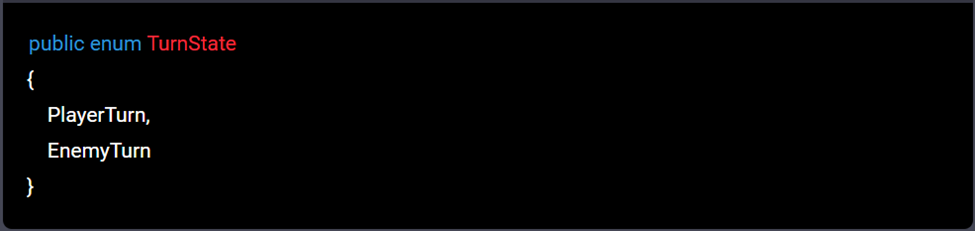
Next, you can create a private variable called turnState of type TurnState, which will hold the current turn state.

Now, instead of checking the boolean isPlayerTurn, you can check the turnState variable to determine what actions to take.

To switch between turn states, you can define a function called ChangeTurnState, which takes a TurnState argument and sets the turnState variable accordingly.

When the player chooses to attack or defend, you can call ChangeTurnState to switch to the enemy's turn.
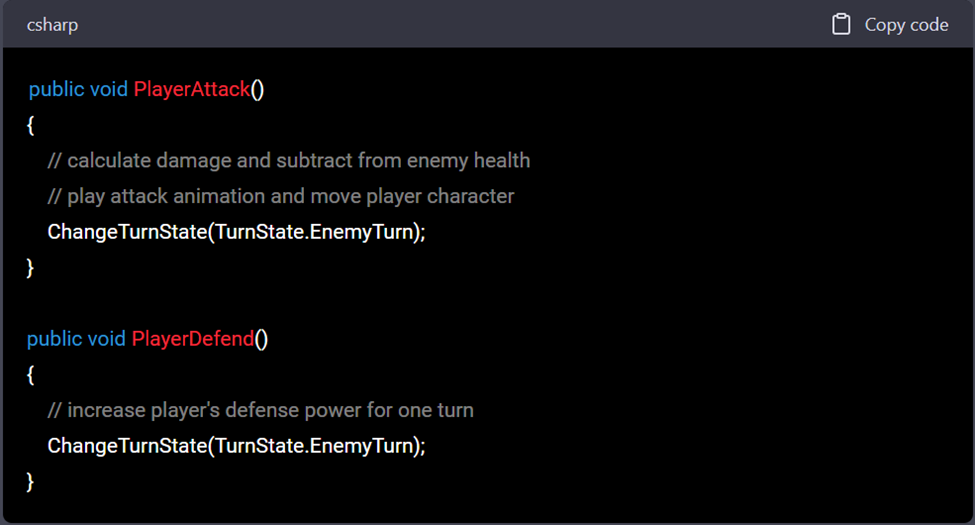
During the enemy's turn, you can call ChangeTurnState to switch back to the player's turn when the turn is complete.
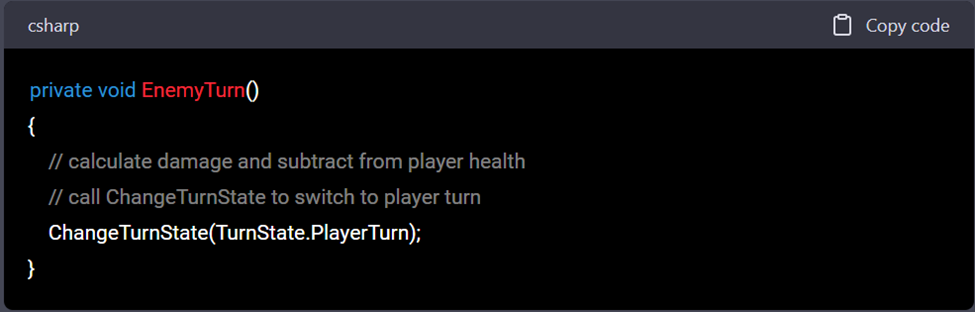
Overall, using an enum to manage turn states can be a cleaner and more flexible solution than using a boolean. By defining all possible states in the TurnState enum, you can easily expand the functionality of your turn-based system in the future if needed.
Animating the Battle Actions
To animate the battle actions, we will use Unity's animator and animation controller. First, we will create an animation controller that contains two states, one for the player attacking and one for the enemy attacking. We will then create animation clips for each state, which will contain the actual animation of the character attacking.
Next, we will create a script that will play the appropriate animation clip when the player or enemy attacks. We will also use the script to move the character toward the target so that it looks like they are physically attacking.
Finally, we will use the animator to transition between the attack and idle states, so that the character returns to their idle animation after the attack is complete.
Conclusion
In this tutorial, we have covered the basics of creating a turn-based battle system in Unity. We started by setting up the scene with game objects and UI elements, and then moved on to creating the battle logic using a turn manager script. Finally, we animated the battle actions using Unity's animator and animation controller. With this foundation, you can now build on this tutorial to create more complex turn-based battle systems.
Wow! Thanks, mate for sharing the brief logic of the game. That is amazing.